Amazon S3 Class for Xojo
Feb 19, 2019 06:37 PM
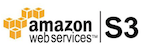
When developing Xojo Web Apps you have several choices to make like what type of database and how to store documents and photos. Storing files in the database results in large databases which are difficult to maintain. Storing files on your Xojo Web App server means keeping an eye on free disk space. Amazon S3 solves all these issues with inexpensive and practically infinite storage. The MBS Plugin has Amazon S3 support, so we created an easy to use Amazon S3 Xojo Class.
Using the S3 Class is easy. First initialize the class then start making calls!
Before getting into the calls, I'd like to have a big thank you to Tim Dietrich and Christian Schmitz for their example code which made putting the S3 Class together super easy. Once you have your Amazon account created, enabled S3 and add an S3 Bucket, I'd suggest using an App like CyberDuck to connect to S3 as a viewer to see the result of making calls to the S3 Class.
Download: Xojo S3 Class
Instantiate
Once you drag the class to your project you can instantiate S3. Just set your Keys, update the Region, Domain, and set your Bucket name.
// Init S3
Dim theS3 As New S3( _
"AccessKey", _
"SecretKey", _
"us-east-1", _
"s3.us-east-1.amazonaws.com", _
"Bucket" _
)
A Note About S3 Keys
S3 uses Keys to reference files and folders. In the List Files example below, we're looking for what is in the "PhotoURL" folder which is in the UUID "F30BB9DD-DE9A-4F13-91B1-79BA4A776585" folder which is in the "Contacts" folder. This how we store files for a specific Contact Photo. In our database we have a Table called "Contacts" that has a record with that UUID and a column called "PhotoURL" which stores the key and the filename that was most recently updated which might look like "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/PhotoOfHal.png". Amazon has a ton of information about S3.
List Files
Get a Listing of Content, technically Files and Folders via a Key as XML. Amazon returns the Listings as XML.
// S3 List as XML
Dim theListXML As String
theListXML = theS3.ListGetXML( "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/" )
Get a Listing of Content, technically Files and Folders via a Key as a Dictionary. Internally, this method calls ListGetXML and transforms the data into a Dictionary for easier processing.
// S3 List as Dictionary
Dim theListDict() as Dictionary
theListDict = theS3.ListGetDict( "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/" )
FolderItem Upload and Download
Upload a FolderItem via a Key. To upload a file, you first define the folder Item and then pass the Key and FolderItem.
Dim theFolderItem as FolderItem = SpecialFolder.Desktop.Child("test-one.png")
Dim theFolderItemUpload as string // 200 is successful
theFolderItemUpload = theS3.FolderItemUpload( "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/" + theFolderItem.Name, theFolderItem )
Download a FolderItem via a Key. To download a file, you first define the folder Item and then pass the Key and FolderItem.
Dim theFolderItem as FolderItem = SpecialFolder.Desktop.Child("test-one.png")
Dim theFolderItemUpload as string // 200 is successful
theFolderItemUpload = theS3.FolderItemDownload( "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/" + theFolderItem.Name, theFolderItem )
String Upload and Download
Upload a String via a Key. Uploading a string is similar to uploading a FolderItem except that the string is held in memory rather than read from a file.
dim theString as string = "Hello World!"
dim theStringUpload as string // 200 is successful
theStringUpload = theS3.StringUpload( "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/StringUpload.txt", theString )
Download a String via a Key. Downloading a string is similar to downloading a FolderItem except that the string is held in memory rather than written to a file.
dim theStringDownload as string // 200 is successful
theStringDownload = theS3.StringDownload( "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/StringUpload.txt" )
Delete
Delete Content via a Key. When run, the file is deleted returning "204" representing "No Content" if successful.
dim theKeyDelete as string // 204 is successful
theKeyDelete = theS3.KeyDelete( "Contacts/F30BB9DD-DE9A-4F13-91B1-79BA4A776585/PhotoURL/StringUpload.txt" )
Questions or Comments
Post on this Xojo Forum Thread.
